Saving salary bands/ranges for JobRelay job
In this article
Most posting providers including Broadbean, LogicMelon and Idibu for example send the salary data for jobs as integers. Usually there is a from and a to value sent over.
Often, on your WordPress site you may want to present a salary band or range to candidates on the frontend of your site, allowing them to see all jobs within a range of salary.
In order to make this work, you need a little bit of extra work on your WordPress site. In this tutorial, I will show you how to setup salary bands on your website and then assign the job to the appropriate salary range, based off of the salary from value.
Create a custom taxonomy for salary range
The salary ranges themselves will be stored as terms in a custom taxonomy on your WordPress site.
There are a number of ways to create a custom taxonomy in WordPress including writing code or using a plugin. In this example we will use a plugin as this is perhaps the easy option.
The plugin we are going to use is Advanced Custom Fields – free to use from the WordPress plugins repository.
Once you have installed the plugin, navigate to ACF > Taxonomies to create your new taxonomy. Add your new taxonomy with the following settings.
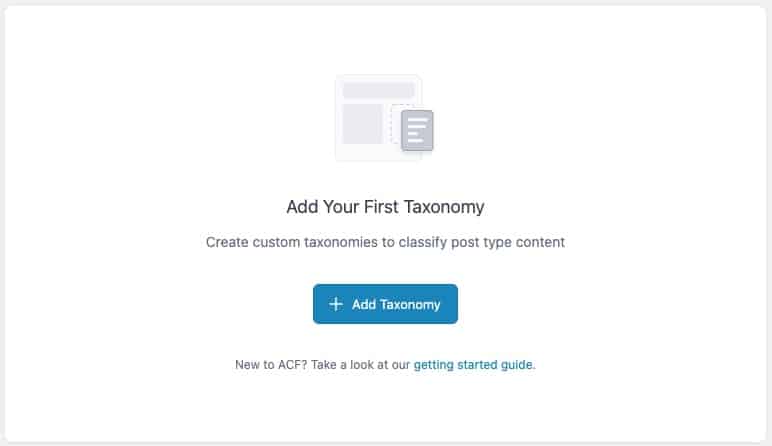
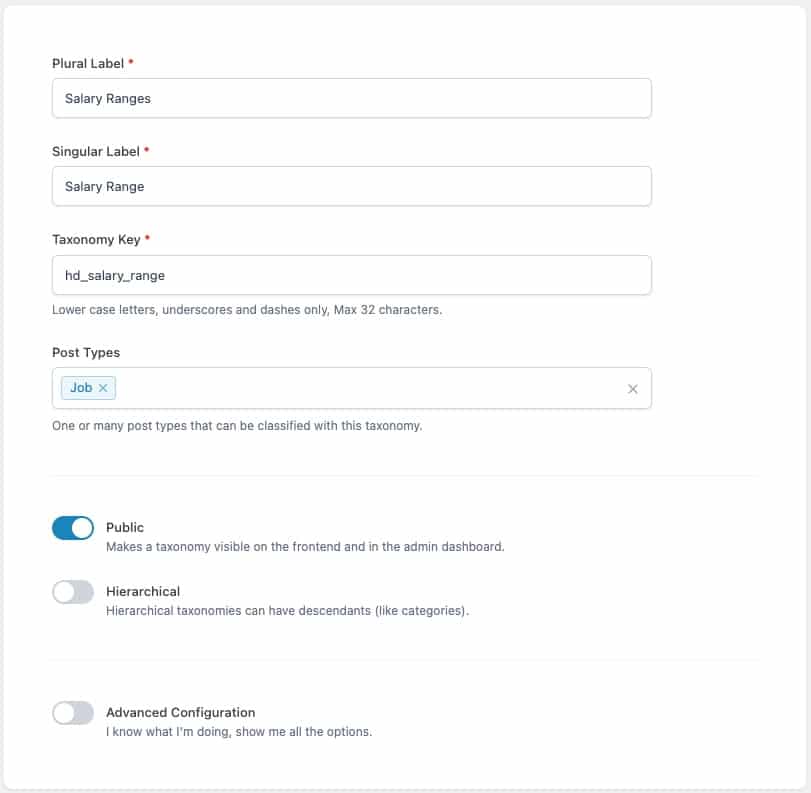
The import thing is to assign your new taxonomy to your jobs post type.
Once this has been done, you should see your new “Salary Ranges” custom taxonomy underneath your jobs post type menu.
Create the salary bandings/ranges as terms in your new taxonomy
Navigate to your custom taxonomy listing screen in WordPress, which should be nested beneath your job custom post type menu item.
Add in your salary ranges like so:
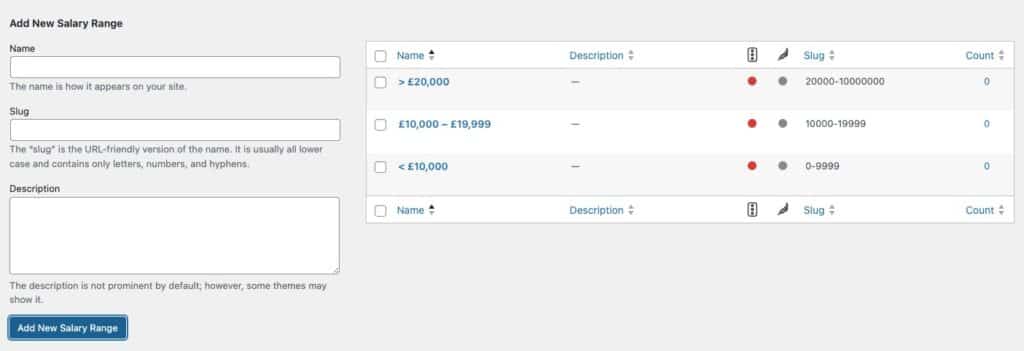
The term name should be the display label of your taxonomy range and thus formatted in the most read format possible. This can include spaces and a currency symbol if you wish.
The important part of the slug, this should form the lower and upper ends of the range. For example, a salary range of < £10,000
would have a slug of 0-10000
. A range of £10,000 - £19,999
would have a slug of 10000-19999
Save the salary range term when a job is delivered from JobRelay
The final step is to save the correct salary range term based on the salary_from
value when the job is sent.
To do this you can use the code below. You can add this code either in your active themes functions.php
file, as part of your own plugin or using a PHP snippets plugin.
<?php
/**
* Assign a salary range term to a job post based on the salary from value.
*
* @param int $post_id The ID of the job post.
* @param array $job_data The job data.
*/
function hd_set_jobrelay_salary_range( $post_id, $job_data ) {
// if we don't have a salary from value.
if ( empty( $job_data['salary_from'] ) ) {
return;
}
// get the salary ranges - the terms from our custom taxonomy.
$salary_ranges = get_terms(
[
'taxonomy' => 'hd_salary_range', // change to your taxonomy name.
'hide_empty' => false
]
);
// if we have salary ranges.
if ( ! empty( $salary_ranges ) && ! is_wp_error( $salary_ranges ) ) {
// loop through each range.
foreach ( $salary_ranges as $salary_range ) {
// split the term slug to get the lower and upper values for the range.
$range_values = explode( '-', $salary_range->slug );
// if the salary from is withing the range of this term.
if ( $job_data['salary_from'] >= $range_values[0] && $job_data['salary_from'] <= $range_values[1] ) {
// set the salary range term for the job.
wp_set_object_terms( $post_id, $salary_range->name, 'hd_salary_range' );
return;
}
}
}
}
add_action( 'jobrelay_wpcon_job_inserted_complete', 'hd_set_jobrelay_salary_range', 10, 2 );
When a new job is sent to your site, it will now be assigned the correct salary range, based on the value sent in the salary_from
field.